Toggle to dynamically change the number of returned results
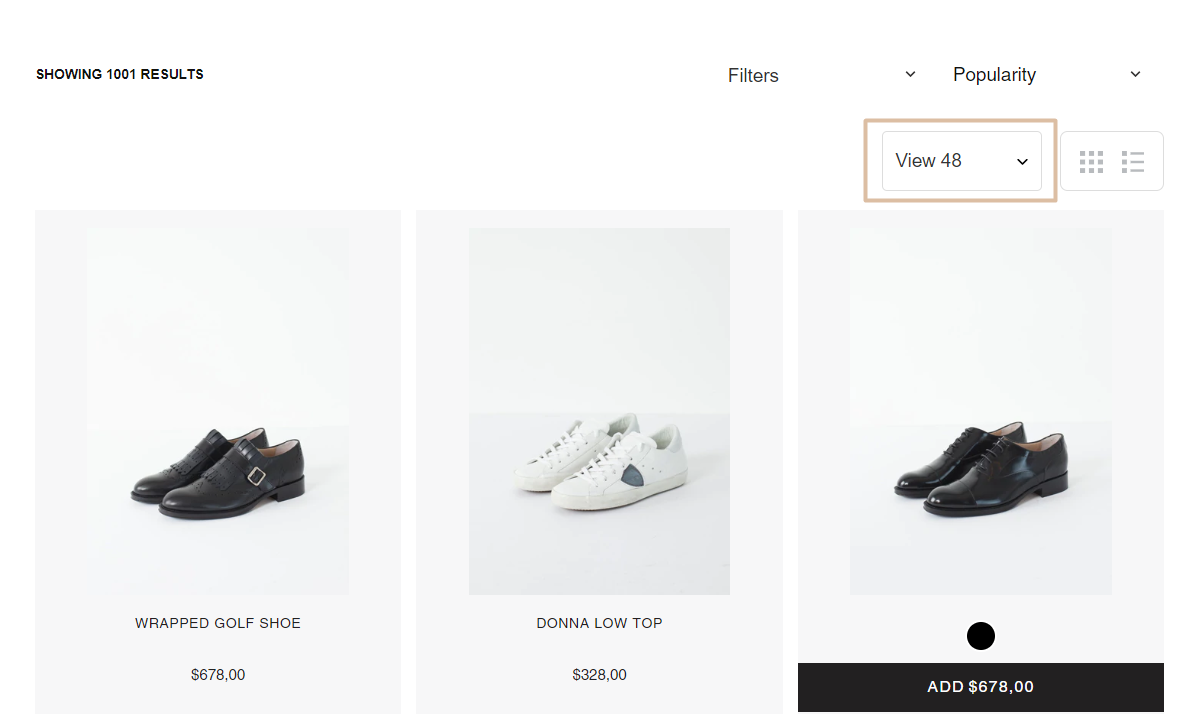
#2. Time Estimates
Set up in Platform: n/a
Integration: 0.5 hour
Styling: 0.5 hour (optional)
#3. Functional Overview
Components:
- any other component connected by
@findify/react-connect
#4. Integration Steps
Here we will be showing how to create a component which will change the number of the returned results. This component can be placed anywhere on the page.
This can be done with the update function
, which is available in every component that is connected via @findify/react-connect
. You can find more about connectors in our documentation or the GitHub repository.
import React, { useState, useEffect } from "react"
import { useItems } from '@findify/react-connect';
// component to change search results quantity
const ViewResultsSelect = () => {
const { update } = useItems();
// state to change the number of search results
const [ dataLimits, changeDataLimit ] = useState(12);
// function to change the number of search results
const onChangeDataLimits = (e) => {
const regexp=/\D/g;
changeDataLimit(e.target.value.replace(regexp, ""));
}
// data update Limits according to state
useEffect(() => {
update('limit', dataLimits)
}, [dataLimits]);
const options = [12, 24, 36, 48].map((item, idx) => {
return <option key={idx}> View { item } </option>
})
return(
<div className="findify-selector-wrapper">
<div className="findify-custom-wrapper">
<select id="findify-custom-selector" onChange={onChangeDataLimits}>
{options}
</select>
</div>
</div>
)
}
export default ViewResultsSelect;
After it's done, all you need is to place this component decide where you want to have it on the page and place it accordingly.
#5. MJS Version
This module has been optimized for MJS version 7.1.37
Updated 6 months ago